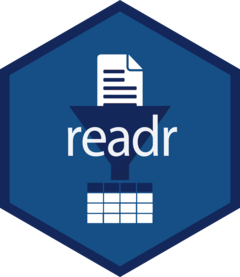
Download PDF
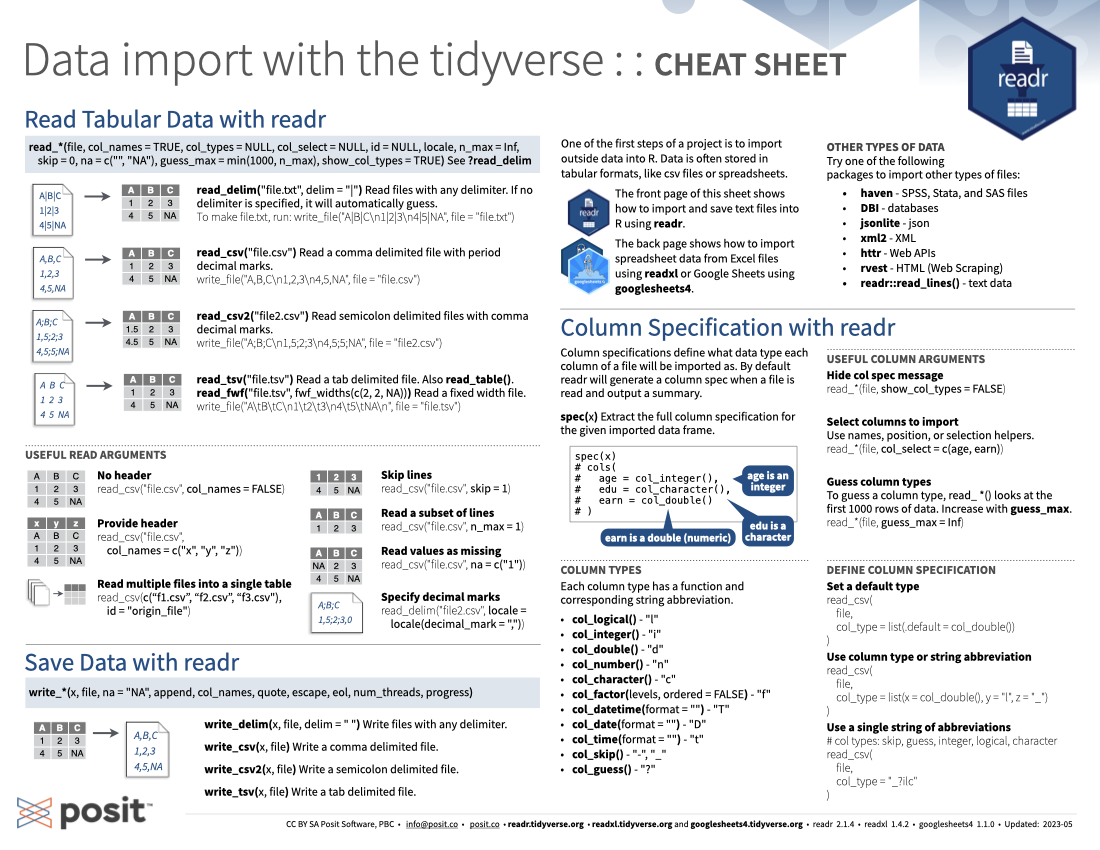
Translations (PDF)
One of the first steps of a project is to import outside data into R. Data is often stored in tabular formats, like csv files or spreadsheets.
For importing other types of data try one of the following packages:
See ?read_delim
.
read_*(
file,
col_names = TRUE, col_types = NULL, col_select = NULL,
show_col_types = TRUE
id = NULL, locale,
n_max = Inf, skip = 0, guess_max = min(1000, n_max),
na = c("", "NA")
)
Read files with any delimiter: read_delim()
. If no delimiter is specified, it will automatically guess.
If the file you want to import is the following:
A|B|C
1|2|3
4|5|NA
Read it with read_delim()
and it will look like the following when imported:
To make file.txt
, run:
Read a comma delimited file with period decimal marks: read_csv()
.
If the file you want to import is the following:
A,B,C
1,2,3
4,5,NA
Read it with read_csv()
and it will look like the following when imported:
To make file.csv
, run:
Read semicolon delimited files with comma decimal marks: read_csv2()
.
If the file you want to import is the following:
A;B;C
1,5;2;3
4,5;5;NA
Read it with read_csv2()
and it will look like the following when imported:
To make file2.csv
, run:
Read a tab delimited file: read_tsv()
or read_table()
.
Read a fixed width file: read_fwf("file.tsv", fwf_widths(c(2, 2, NA)))
.
If the file you want to import is the following:
A B C
1 2 3
4 5 NA
Read it with read_tsv()
and it will look like the following when imported:
To make tsv
, run:
Suppose you have the following CSV files that you want to read in, called file.csv
:
file.csv
A,B,C
1,2,3
4,5,NA
file3.csv
A,B,C
7,8,9
NA,11,12
To make these files, run:
No header: col_names = FALSE
Provide header: col_names = c("x", "y", "z")
Skip lines:
Read a subset of lines:
Read values as missing:
Specify decimal marks:
Read multiple files into a single table:
write_delim(x, file, delim = " ")
write_csv(x, file)
write_csv2(x, file)
write_tsv(x, file)
Column specifications define what data type each column of a file will be imported as. By default readr will generate a column spec when a file is read and output a summary.
spec(df)
: Extract the full column specification for the given imported data frame.
Each column type has a function and corresponding string abbreviation.
col_logical() - "l"
col_integer() - "i"
col_double() - "d"
col_number() - "n"
col_character() - "c"
col_factor(levels, ordered = FALSE) - "f"
col_datetime(format = "") - "T"
col_date(format = "") - "D"
col_time(format = "") - "t"
col_skip() - "-", "_"
col_guess() - "?"
Hide col spec message:
Select columns to import: Use names, position, or selection helpers.
Guess column types: To guess a column type, read_ *()
looks at the first 1000 rows of data. Increase with guess_max
.
Set a default type:
Use column type or string abbreviation:
Use a single string of abbreviations:
Read a .xls or .xlsx file based on the file extension, e.g. read_excel("excel_file.xlsx")
. See Useful read arguments for more read arguments. Also read_xls()
and read_xlsx()
.
If the Google sheet you want to import is the following:
A | B | C | D | E |
---|---|---|---|---|
x1 | x2 | x3 | x4 | x5 |
x | z | 8 | ||
y | 7 | 9 | 10 |
It will look like the following when imported:
Specify which sheet to read by position or name: read_excel(path, sheet = NULL)
read_excel(path, sheet = 1)
read_excel(path, sheet = "s1")
Get a vector of sheet names: excel_sheets(path)
excel_sheets("excel_file.xlsx")
To read multiple sheets:
Get a vector of sheet names from the file path.
Set the vector names to be the sheet names.
Use purrr::map()
and purrr::list_rbind()
to read multiple files into one data frame.
Column specifications define what data type each column of a file will be imported as.
Use the col_types
argument of read_excel()
to set the column specification.
Guess column types: To guess a column type, read_excel()
looks at the first 1000 rows of data. Increase with the guess_max
argument.
Set all columns to same type, e.g. character:
Set each column individually:
Column types:
logical | numeric | text | date | list |
---|---|---|---|---|
TRUE | 2 | hello | 1947-01-08 | hello |
FALSE | 3.45 | world | 1956-10-21 | 1 |
skip
guess
logical
date
numeric
text
Use list
for columns that include multiple data types. See tidyr and purrr for list-column data.
Read a sheet from a URL, a Sheet ID, or a dribble samefrom the googledrive package. See Useful read arguments for more read arguments.
Same as range_read()
.
If the Google sheet you want to import is the following:
A | B | C | D | E |
---|---|---|---|---|
x1 | x2 | x3 | x4 | x5 |
x | z | 8 | ||
y | 7 | 9 | 10 |
It will look like the following when imported:
URLs are in the form:
https://docs.google.com/spreadsheets/d/
SPREADSHEET_ID/edit#gid=SHEET_ID
Get spreadsheet meta data: gs4_get(ss)
Get data on all spreadsheet files: gs4_find(...)
Get a tibble of properties for each worksheet: sheet_properties(ss)
. Also sheet_names()
.
write_sheet(data, ss = NULL, sheet = NULL)
: Write a data frame into a new or existing Sheet. gs4_create(name, ..., sheets = NULL)
: Create a new Sheet with a vector of names, a data frame, or a (named) list of data frames.sheet_append(ss, data, sheet = 1)
: Add rows to the end of a worksheet.Column specifications define what data type each column of a file will be imported as.
Use the col_types
argument of read_sheet()
/range_read()
to set the column specification.
Guess column types: To guess a column type read_sheet()
/range_read()
looks at the first 1000 rows of data. Increase with guess_max
.
Set all columns to same type, e.g. character:
Set each column individually:
Column types:
skipped my lunch 🥙 🍱 and: “_” or “-”
guess: “?”
logical: “l”
integer: “i”
double: “d”
numeric: “n”
date: “D”
datetime: “T”
character: “c”
list-column: “L”
cell: “C” (returns list of raw cell data)
Use list for columns that include multiple data types. See tidyr and purrr for list-column data.
Use the range argument of readxl::read_excel() or googlesheets4::read_sheet() to read a subset of cells from a sheet.
Also use the range argument with cell specification functions cell_limits()
, cell_rows()
, cell_cols()
, and anchored()
.
CC BY SA Posit Software, PBC • info@posit.co • posit.co
Learn more at
Updated: 2024-05.
[1] '2.1.5'
[1] '1.4.3'
[1] '1.1.1'