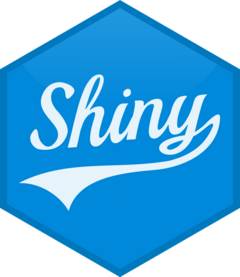
Download PDF
A Shiny app is an interactive web page (ui) powered by a live Python session run by a server (or by a browser with Shinylive).
Users can manipulate the UI, which will cause the server to update the UI’s displays (by running Python code).
Save your app as app.py
in a directory with the files it uses.
Run shiny create .
in the terminal to generate an app.py
file based on a template.
Launch apps with shiny run app.py --reload
from shiny import App, render, ui
import matplotlib.pyplot as plt
import numpy as np
# Nest Python functions to build an HTML interface
app_ui = ui.page_fluid( # Layout the UI with Layout Functions
# Add Inputs with ui.input_*() functions
ui.input_slider(
"n", "Sample Size", 0, 1000, 20
),
# Add Outputs with ui.ouput_*() functions
ui.output_plot("dist")
)
def server(input, output, session):
# For each output, define a function that generates the output
@render.plot # Specify the type of output with a @render. decorator
def dist(): # Use the output id as the function name
# Call the values of UI inputs with input.<id>()
x = np.random.randn(input.n())
plt.hist(x, range=[-3, 3])
# Call App() to combine app_ui and server() into an interactive app
app = App(app_ui, server)
Share your app in three ways:
Host it on shinyapps.io, a cloud based service from Posit. To deploy Shiny apps:
rsconnect deploy shiny <path to directory>
Purchase Posit Connect, a publishing platform for R and Python. posit.co/products/enterprise/connect/
Use open source deployment options. shiny.posit.co/py/docs/deploy.html
Shinylive apps use WebAssembly to run entirely in a browser–no need for a special server to run Python.
shinylive export myapp site
. Then deploy to a hosting site like Github or Netifly.To embed a Shinylive app in a Quarto doc, include the below syntax.
Match ui.output_* functions to @render.* decorators
decorator | function |
---|---|
@render.data_frame |
ui.output_data_frame(id) |
@render.image |
ui.output_image(id, width, height, click, dblclick, hover, brush, inline) |
@render.plot |
ui.output_plot(id, width, height, click, dblclick, hover, brush, inline) |
@render.table |
ui.output_table(id) |
@render.text |
ui.output_text(id, container, inline) also ui.output_text_verbatim() |
@render.ui |
ui.output_ui(id, inline, container, ...) also ui.output_html() |
@sessiom.download |
ui.output_download_button(id, label, icon,...) |
Use a ui.input_*()
function to make an input widget that saves a value as input.<id>
. Input values are reactive and need to be called as input.<id>()
.
ui.input_action_button(id, label, icon, width, ...)
ui.input_action_link(id, label, icon, ...)
ui.input_checkbox(id, label, value, width)
ui.input_checkbox_group(id, label, choices, selected, inline, width)
ui.input_date(id, label, value, min, max, format, startview, weekstart, language, width, autoclose, datesdisabled, daysofweekdisabled)
ui.input_date_range(id, label, start, end, min, max, format, startview, weekstart, language, separator, width, autoclose)
ui.input_file(id, label, multiple, accept, width, buttonLabel, placeholder, capture)
ui.input_numeric(id, label, value, min, max, step, width)
ui.input_password(id, label, value, width, placeholder)
ui.input_radio_buttons(id, label, choices, selected, inline, width)
ui.input_select(id, label, choices, selected, multiple, selectize, width, size) Also ui.input_selectize()
ui.input_slider(id, label, min, max, value, step, ticks, animate, width, sep, pre, post, timeFormat, timezone, dragRange)
ui.input_switch(id, label, value, width)
ui.input_text(id, label, value, width, placeholder, autocomplete, spellcheck) Also ui.input_text_area()
Reactive values work together with reactive functions. Call a reactive value from within the arguments of one of these functions to avoid the error No current reactive context
.
ui.input_*()
@reactive.file_reader()
@reactive.poll()
@reactive.Effect
reactive.invalidate_later()
reactive.Calc()
reactive.isolate()
@reactive.event()
@render*
ui.input_*()
makes an input widget that saves a reactive value as input.<id>()
.
reactive.value( )
Creates an object whose value you can set.
@reactive.calc
makes a function a reactive expression. Shiny notifies functions that use the expression when it becomes invalidated, triggering recomputation. Shiny caches the value of the expression while it is valid to avoid unnecessary computation.
@reactive.event()
Makes a function react only when a specified value is invalidated, here input.a
.
ui.output_*()
adds an output element to the UI.
@render.*
decorators render outputs
def <id>():
A function to generate the output
@reactive.effect
Reactively trigger a function with a side effect. Call a reactive value or use @reactive.event
to specify when the function will rerun.
reactive.isolate()
Create non-reactive context within a reactive function. Calling a reactive value within this context will not cause the calling function to re-execute should the value become invalid.
Combine multiple elements into a “single element” that has its own properties with a panel function:
ui.panel_absolute()
ui.panel_conditional()
ui.panel_fixed()
ui.panel_main()
ui.panel_sidebar()
ui.panel_title()
ui.panel_well()
ui.row() / ui.column()
Layout panels with a layout function. Add elements as arguments of the layout functions.
ui.page_sidebar()
ui.layout_columns()
with `ui.card()Use ui.nav_panel
with ui.page_navbar
to create tabs:
Use the shinyswatch package to add existing bootstrap themes to your Shiny app ui.
Shiny for Python is quite similar to Shiny for R with a few important differences:
input.<id>()
R
Python
def <id>():
R
Python
@reactive.calc
R
Python
@reactive.effect
R
Python
@reactive.event
R
Python
reactive.Value()
instead of reactiveVal()
R
Python
nav_*()
instead of *Tab()
R
Python
R
Python
CC BY SA Posit Software, PBC • info@posit.co • posit.co
Learn more at shiny.posit.co/py/
Updated: 2024-05.
Version: Shiny 0.10.2