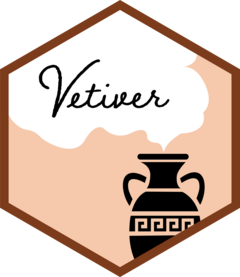
Create a Plumber API to predict with a deployable vetiver_model()
object
Source: R/api.R
vetiver_api.Rd
Use vetiver_api()
to add a POST endpoint for predictions from a
trained vetiver_model()
to a Plumber router.
Usage
vetiver_api(
pr,
vetiver_model,
path = "/predict",
debug = is_interactive(),
...
)
vetiver_pr_post(
pr,
vetiver_model,
path = "/predict",
debug = is_interactive(),
...,
check_prototype = TRUE,
check_ptype = deprecated()
)
vetiver_pr_docs(pr, vetiver_model, path = "/predict", all_docs = TRUE)
Arguments
- pr
A Plumber router, such as from
plumber::pr()
.- vetiver_model
A deployable
vetiver_model()
object- path
The endpoint path
- debug
TRUE
provides more insight into your API errors.- ...
Other arguments passed to
predict()
, such as predictiontype
- check_prototype
Should the input data prototype stored in
vetiver_model
(used for visual API documentation) also be used to check new data at prediction time? Defaults toTRUE
.- check_ptype
- all_docs
Should the interactive visual API documentation be created for all POST endpoints in the router
pr
? This defaults toTRUE
, and assumes that all POST endpoints use thevetiver_model()
input data prototype.
Details
You can first store and version your vetiver_model()
with
vetiver_pin_write()
, and then create an API endpoint with vetiver_api()
.
Setting debug = TRUE
may expose any sensitive data from your model in
API errors.
Several GET endpoints will also be added to the router pr
, depending on the
characteristics of the model object:
a
/pin-url
endpoint to return the URL of the pinned modela
/metadata
endpoint to return any metadata stored with the modela
/ping
endpoint for the API healtha
/prototype
endpoint for the model's input data prototype (usecereal::cereal_from_json()
) to convert this back to a vctrs ptype
The function vetiver_api()
uses:
vetiver_pr_post()
for endpoint definition andvetiver_pr_docs()
to create visual API documentation
These modular functions are available for more advanced use cases.
Examples
cars_lm <- lm(mpg ~ ., data = mtcars)
v <- vetiver_model(cars_lm, "cars_linear")
library(plumber)
pr() %>% vetiver_api(v)
#> # Plumber router with 4 endpoints, 4 filters, and 1 sub-router.
#> # Use `pr_run()` on this object to start the API.
#> ├──[queryString]
#> ├──[body]
#> ├──[cookieParser]
#> ├──[sharedSecret]
#> ├──/logo
#> │ │ # Plumber static router serving from directory: /home/runner/work/_temp/Library/vetiver
#> ├──/metadata (GET)
#> ├──/ping (GET)
#> ├──/predict (POST)
#> └──/prototype (GET)
#>
## is the same as:
pr() %>% vetiver_pr_post(v) %>% vetiver_pr_docs(v)
#> # Plumber router with 4 endpoints, 4 filters, and 1 sub-router.
#> # Use `pr_run()` on this object to start the API.
#> ├──[queryString]
#> ├──[body]
#> ├──[cookieParser]
#> ├──[sharedSecret]
#> ├──/logo
#> │ │ # Plumber static router serving from directory: /home/runner/work/_temp/Library/vetiver
#> ├──/metadata (GET)
#> ├──/ping (GET)
#> ├──/predict (POST)
#> └──/prototype (GET)
#>
## for either, next, pipe to `pr_run()`